Is numpy array row or column vector
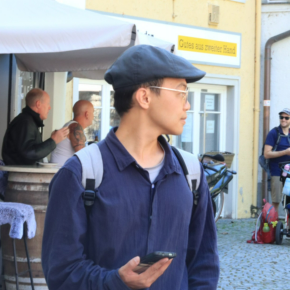
tldr: it is row vector, just automatically expanded/converted when doing matrix multiplication with the proper dimension.
I was constantly bothered by the question of “is numpy array row or column vector”? I know for a single dimensional vector, it is neither row nor column, as already pointed out by others . But without a proper understanding of this question, I’m not confident enough everytime I try to imageine what would happen when I’m implementing my own algebra, and this unconfidence is really annoying cause I will always be anxious that I might be writing something wrong.
I view this question as, when a 2-D numpy array(which is a matrix) and a 1-D numpy array multiplies, in which dimension of that 2-D numpy array does the matrix multiplication happen. This is not trivial since both side can work in theory but they yield completely different results. So today I’m using simple python interactive shell to solve this question in one fell swoop.
Simple experiment
Define a matrix m
:
1 | >>> m = np.array([[1,2,3],[2,3,4],[3,4,5]]) |
Define a vector v
:
1 | >>> v = np.array([1,2,3]) |
Doing element-wise multiplication:
1 | >>> m * v |
This is quiet intuitive to understand: all the smallest element (a 3 dim array) in both components are multiplied element-wise.
Doing a matrix multiplication directly:
1 | >>> m @ v |
It is interesting that in both direction they have the same result. Because commutative rule doesn’t apply to matrix multiplication, not only shouldn’t m @ v
and v @ m
have the same output, but also at least one side should not be valid operation. So the result suggests that an implicit conversion happened in the numpy operation @.
This kind of implicit conversion is both smart and dumb — smart in the sense that it makes the interpreter inference the shape so that they can guess what you want to write. But that also means if you made a logic mistake in your code and happens to be inferenced properly, the interpreter will perceed without throwing error and don’t give you proper warning even if you were doing the wrong computation. It also the culprit hinders me to be confident on what is really happening underneath.
Eliminate the implicit conversion
So in order to eliminate space for such ambiguity, we just manually boardcast the vector to the same 2-d array and see what happens. As expected, this time we can see how they are treated differently:
1 | >>> v[None,:] |
The other side works the same as well:
1 | >>> v[:,None] |
Conclusion
So finally the answer is clear, a single vector is at its core treated as a row vector in the matrix. No need to worry, the numpy world now is perfectly ordered in my mind ;). I would wish such implicit conversion were never implemented in the first place, then I might learn this fact way earlier with just a few runtime errors.
- Title: Is numpy array row or column vector
- Author: Lumen Yang
- Created at : 2024-02-05 15:42:22
- Updated at : 2024-02-11 09:22:30
- Link: https://www.lumeny.io/2024/02/05/Is numpy array row or column vector/
- License: This work is licensed under CC BY-NC-SA 4.0.